Introducing: My GraphQL Go Server
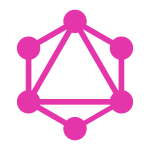
I’m excited to share my hobby project from the last few months: a GraphQL server library for Go. While it doesn’t implement the whole spec and might not be ready for production, I think it’s in a useful enough state to share more broadly. I’ve tested this library out with some toy projects and the results have been promising: I’m able to quickly publish a Go struct as a GraphQL endpoint.
Check it out now by reading the docs and adding it to your project with:
go get zombiezen.com/go/graphql-server/graphql
The Hello World example:
package main
import (
"log"
"net/http"
"zombiezen.com/go/graphql-server/graphql"
"zombiezen.com/go/graphql-server/graphqlhttp"
)
// Query is the GraphQL object read from the server.
type Query struct {
Greeting string
}
func main() {
// Set up the server.
schema, err := graphql.ParseSchema(`
type Query {
greeting: String!
}
`, nil)
if err != nil {
log.Fatal(err)
}
queryObject := &Query{Greeting: "Hello, World!"}
server, err := graphql.NewServer(schema, queryObject, nil)
if err != nil {
log.Fatal(err)
}
// Serve over HTTP using NewHandler.
http.Handle("/graphql", graphqlhttp.NewHandler(server))
http.ListenAndServe(":8080", nil)
}
There are already a few other Go GraphQL libraries out there, so why did I write my own? In short, I wasn’t satisfied with the support for accessing the selection set from resolver functions, meaning that servers can’t optimize out unused queries. I also wanted to decouple from JSON serialization to make servers easier to test. For more technical details, see the comparison in the README.
This library is still in beta, so early feedback is appreciated! Give it a try and let me know what you think. See the CONTRIBUTING guide for details on how you can get involved.